The object or entity is the center of the object-oriented programming paradigm. Both developers and programmers have made great strides in object-oriented programming. Multi-billion dollar companies have profited from optimizing their systems and processes for their employees and customers. However, this requires devoting a significant amount of time to learning computer languages and coding techniques.
A novice programmer often has a lot of homework and lots of assignments to complete at college or university. Luckily, there are online resources like call-tracking.org that allow you to hire experts to help you with your programming homework and more.
1. Class Concept
Class is the first core idea in object-oriented programming. A class is an abstract structure that provides two perspectives on real-world objects: its attributes (properties) and methods (the actions it can perform or its behavior).
Employees, for instance, can be represented as a class in object-oriented programming. In this case, the class Employees represents all of the employees, and its properties include surname, first name, address, and date of birth. Operations on employees include changing their salaries, going on leave, retiring, etc.
All class instances are referred to as objects and are created from the Class through the instantiation process. The Class is ultimately a mold or template. Every object is a class instance as a result.
Three unique methods are used during the instantiation of a class, and understanding these methods is crucial.
Constructor
There are three different kinds of builders:
The copy constructor (or copy constructor) takes a single argument of the same type as the object to be created (typically in the form of a constant reference), copies the attributes from the object passed in the argument to the object to be created, and is called by default when an object is created (offered by default during compilation if there is no declared constructor).
If the constructor’s signature matches, the parametric constructor is invoked.
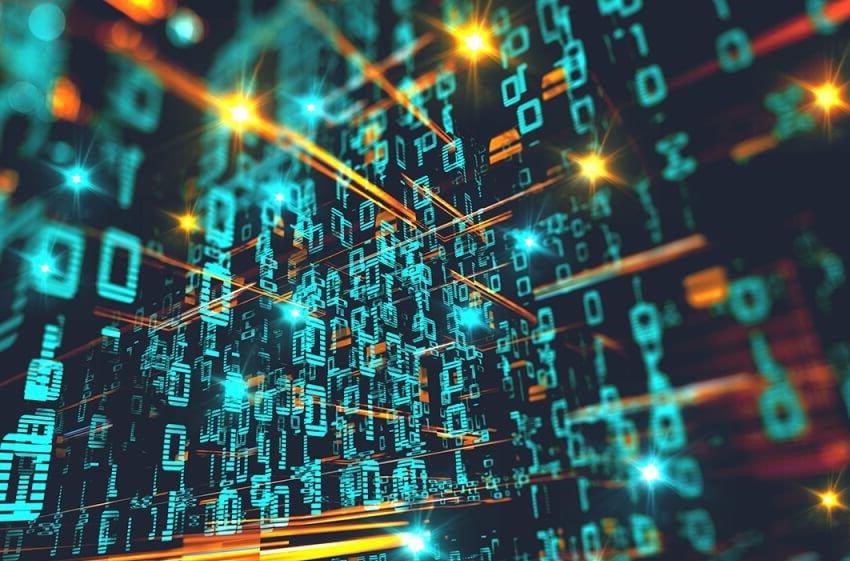
(Get) Accessors and Mutators (set)
These special methods let you access a class’s properties and change them outside, much like an API. They enable the functionalities of the Class to be “called” from the outside.
Without explicitly gaining access to the properties of a class instance, accessors let you retrieve their values from the outside. By preventing its alteration, they secure the property in this way. The mutators provide you the ability to change the value of the properties while verifying that the value you wish to assign to the feature complies with the semantic restrictions placed on the Class.
Destructor
The class instance’s life is ended by this method. It may be called either explicitly or implicitly when the object is erased.
2. Object Concept
The object is the second-most significant concept in object-oriented programming. An object is an instance of a class, as we previously stated. The item resembles a house constructed according to a certain plan in several ways. As long as designers follow this blueprint, all of their homes will be the same.
Technically, an object has three characteristics:
· a name, address, or reference that clearly identifies the object as having that identity;
· specifies that each object has a default value for each of its properties (when specified at instantiation). The states of the object are these values;
· Each object has a set of methods that it can use to carry out any declared actions or behaviours. In OOP, these activities are concretely expressed as methods. Calls to these methods or messages sent by other objects start the potential activities on an object.
3. The Encapsulation Concept
Encapsulation is the third principle of object-oriented programming.
Only the properties of objects are accessible through its methods. Since the methods and attributes that enable altering the objects regardless of their states are contained within the Class, this is possible.
The encapsulation inhibits object alteration outside of its methods and limits direct access to the states. For instance, if you have a Car class and want to set the color property’s value to blue, you must use a method like “define the color” that was put in place by the Class developer. The varied color values can be limited using this technique.
Encapsulation is a system that stops access to or change of items using methods other than those that are suggested. The items’ integrity is ensured by it.
4. The Inheritance Concept
The fourth fundamental idea in object-oriented programming is inheritance. It is an OOP concept that describes how a class can take on traits (such as attributes and methods) from another class.
Classes have parent classes from which their objects can inherit properties. For instance, we may create a Manager class, which is a specialized class of Employee and inherits its properties.
In OOP, inheritance has two key benefits:
· Reuse: You don’t have to create the same Class each time for each specialized Class;
· Specialization: A new class reuses the characteristics and methods of a class by adding operations particular to the new Class.
5. Polymorphism concept
Polymorphism is the final fundamental idea in object-oriented programming. If an object-oriented language can recognize an object as an instance of several different classes at once, it is polymorphic. For instance, Java is a polymorphic language.
Conclusion
Since object-oriented programming is the basis of many high-level programming languages, developers should have a firm grasp of it. By applying the core OOP concepts to understand how simple programs work, you can find the root causes of bottlenecks and remove them by developing more inventive code. Coding suites, learning new languages, and a grasp of OOP ideas can all help you improve your skills.